What is Entity Framework?
In .NET applications (desktop and web), ADO.NET is an old and powerful method for accessing databases and requires a lot of coding. Simply put, all of the work is done manually. Microsoft has therefore introduced Entity Framework to automate the database technique.
Entity Framework (EF), a Microsoft-supported open-source ORM (Object-Relational Mapper) for .NET applications, is a set of utilities and mechanisms that works towards optimizing data-driven applications. The EF fits between the business entities (domain classes) and the database. Additionally, it retrieves data from the database and converts it to business entity objects automatically, and also saves data stored in business entity properties.
Entity Framework Interview Questions for Freshers
1. Explain the advantages of the Entity Framework.
Entity Framework has the following advantages:
- With its excellent prototypes, it is possible to write object-oriented programs.
- By allowing auto-migration, it is simple to create a database or modify it.
- It simplifies the developer's job by reducing the code length with the help of alternate commands.
- It reduces development time, development cost, and provides auto-generated code.
- A unique syntax (LINQ / Yoda) is provided for all object queries, whether they are databases or not.
- It enables the mapping of multiple conceptual models to a single storage schema.
- Business objects can be mapped easily (with drag & drop tables).
2. Describe some of the disadvantages of the Entity Framework.
Entity Framework has the following disadvantages:
- If the developer does not use raw SQL codes, things can become complicated sometimes.
- It is a slower form of the Object Relational Mapper.
- For a big domain model, it's not ideal.
- Some RDMS do not offer this feature.
- EF's main drawback is its lazy loading
- This requires a non-traditional approach to handling data that isn't available for every database.
- Since the data migration functionality is weak, it isn't fully effective in practice.
3. What are the features of the Entity Framework?
Below are some of Entity Framework's basic features:
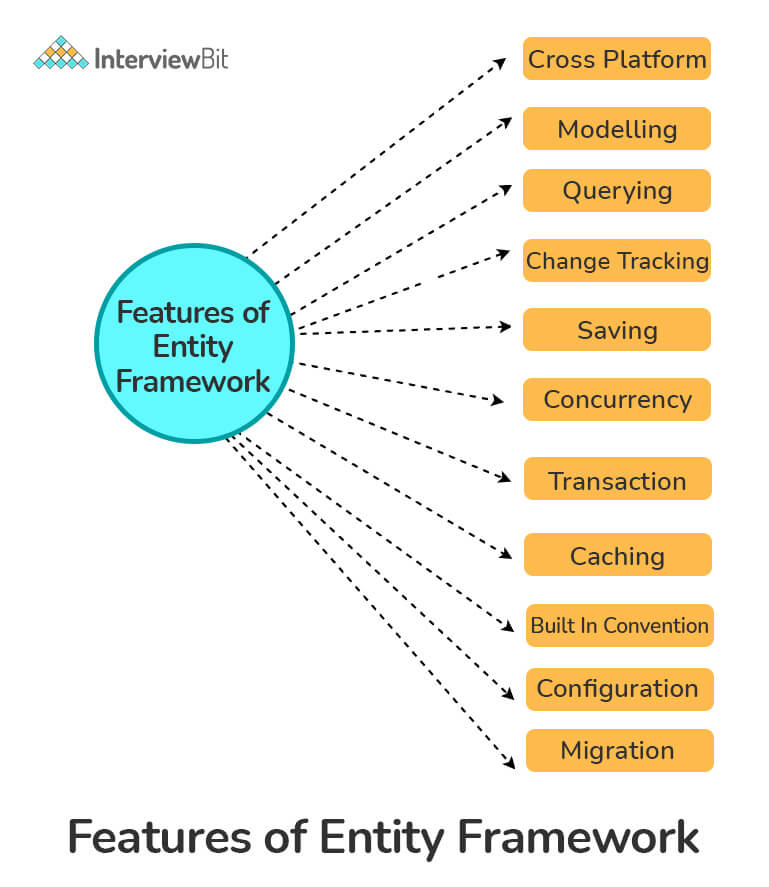
- Cross-Platform: It is lightweight, extensible, open-source, and can be used on Windows, Linux, and Mac.
- Querying: It allows us to retrieve data from underlying databases using LINQ queries, which are then transformed into database-specific query languages.
- Modeling: EDMs (Entity Data Models) are typically created based on POCOs (Plain Old CLR Objects), which are entities with get/set properties of different types. This model is used when querying and saving entity data to the underlying database.
- Change Tracking: By using the SaveChanges method of the context, EF tracks changes to entities and their relationships and ensures the correct updates are performed on the database. Change tracking is enabled by default in EF but can be disabled by setting the AutoDetectChangesEnabled property of DbContext to false.
- Saving: Upon calling the "SaveChanges()" method, EF executes the INSERT, UPDATE, and DELETE commands to the database based on the changes made to entities. "SaveChangesAsync()" is another asynchronous method provided by EF.
- Concurrency: EF provides built-in support for Optimistic Concurrency to prevent an unknown user from overwriting data from the database.
- Transaction: EF's transaction management capabilities automate the querying and saving of data. Furthermore, you can customize the way that transactions are managed.
- Caching: First-level caching of entities is supported out of the box in the EF. Repeated queries will retrieve data from the cache rather than the database in this case.
- Built-in Conventions: EF conforms to the conventions of configuration programming and has a set of default settings that automatically configure the model.
- Configuration: By using the data annotation attribute or Fluent API, we can configure the EF model and override the default conventions.
- Migrations: EF provides migration commands that are executable on the command-line interface or NuGet Package Manager Console to incrementally update the database schema to keep it in sync with the application's data model.
4. Explain different parts of the entity data model.
The Entity Data Model consists of 3 core components that form the basis for Entity Framework. The three main components of EDM are as follows:
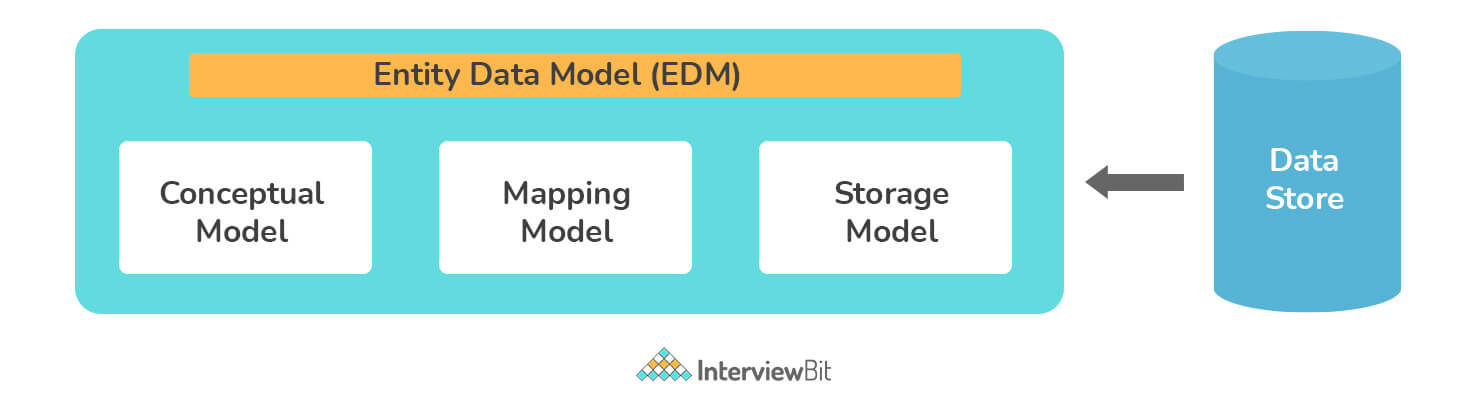
- Conceptual Model: It is also referred to as the Conceptual Data Definition Language Layer (C-Space). Typically, it consists of model classes (also known as entities) and their relationships. Your database table design will not be affected by this. It makes sure that business objects and relationships are defined in XML files.
- Mapping Model: It is also referred to as the Mapping Schema Definition Language layer (C-S Space). Information about how the conceptual model is mapped to the storage model is usually included in this model. In other words, this model enables the business objects and relationships defined at the conceptual layer to be mapped to tables and relationships defined at a logical layer.
- Storage Model: It is also referred to as the Store Space Definition Language Layer (S-Space). Schematically, it represents the storage area in the backend. Therefore, the storage model is also known as a database design model that is composed of tables, keys, stored procedures, views, and related relationships.
5. Explain what the .edmx file contains.
Entity Framework Designer is used to view and edit models stored and created in EDMX files (.edmx extensions). Using the EDMX file, you automatically generate classes that you can interact with within your application.
EDMX files represent conceptual models, storage models, and their mappings. This file contains all the mapping information between SQL tables and objects. In addition, it also includes essential information required for rendering models graphically with ADO.NET Entity Data Designer. Furthermore, it is divided into three divisions, CSDL, MSL, and SSDL.
6. What do you mean by migration? Write its type.
Migration is a tool that was introduced in EF to update the database schema automatically when a model is modified without losing any data or other objects. Migrate Database To Latest Version is a new database initializer used by it. Entity Framework offers two types of migration:
- Automated Migration: Entity Framework 4.3 was the first to introduce automated migration so you don't have to manually migrate databases every time you alter a domain class. For example, you must also change the domain classes for each time you make a change, but with automated migration, you can simply run a command through the Package Manager Console.
- Code-based Migration: When you use a code-based migration, you can configure additional aspects of the migration, like setting the default value of a column, configuring a computed column, etc.
7. What are different types of Entity framework approaches?
Three different approaches to implement Entity Framework are as follows:
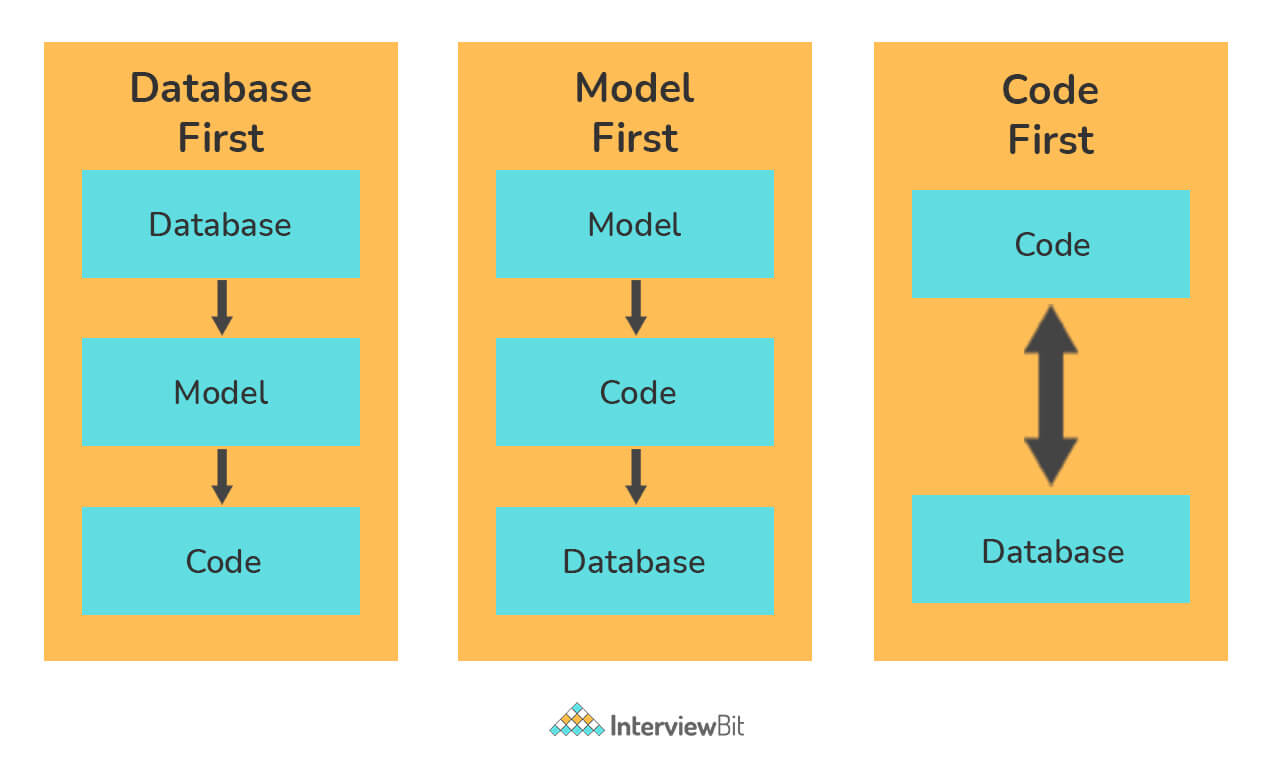
- Code First Approach: The Code First approach primarily uses classes to create the model and its relations, which are then used to create a database. This way, developers can work in an object-oriented manner without considering the database structure. By following this model, developers first write POCO classes and then use these classes to create the database. Code First is the method used by most developers using Domain-Driven Design (DDD).
- Model First Approach: In contrast, the Model First approach uses ORM to build model classes and their relationships. Following the successful creation of the model classes and relationships, the physical database is created using these models.
- Database-First Approach: In Entity Framework, Database First approach is used to build entity models based on existing databases and reduce the amount of code required. By using this approach, domain and context classes can be created based on existing classes.
8. Which according to you is considered the best approach in Entity Framework?
It is impossible to define one approach as the optimal approach when using the Entity Framework. Project requirements and the type of project determine which development approach should be used.
- Database First is a good approach if there is a database present.
- Model First is the optimal choice if no database and model classes exist.
- As long as the domain classes are available, the Code First method is the best choice.
10. What are different entity states in EF?
There are five possible states where an entity can exist:
- Added: It is a state in which an entity exists within the context but does not exist within the database. When the user invokes the SaveChanges method, DbContext usually generates an INSERT SQL query to insert the data into the database. Upon successful completion of the SaveChanges method, the entity's state changes to unchanged.
- Deleted: This state indicates that the entity is marked for deletion has not been removed from the database. Also, it indicates the existence of the entity in the database. When the user invokes the SaveChanges method, DbContext usually generates a DELETE SQL query to delete or remove the entity from the database. Upon successful completion of the delete operation, DbContext removes the entity.
- Modified: When the entity is modified, its state becomes Modified. Also, it indicates the existence of the entity in the database. When the user invokes the SaveChanges method, DbContext usually generates an UPDATE SQL query to update the entity from the database. Upon successful completion of the SaveChanges method, the entity's state changes to unchanged.
- Unchanged: The entity is being tracked by the context and exists in the database, and its property values have not changed from the values in the database
- Detached: This state indicates that the entity is not tracked by the DbContext.
11. Write the importance of the T4 entity in Entity Framework.
In Entity Framework code generation, T4 files are crucial. EDMX XML files are read by T4 code templates, which generate C# behind code. The generated C# behind code consists only of your entity and context classes.
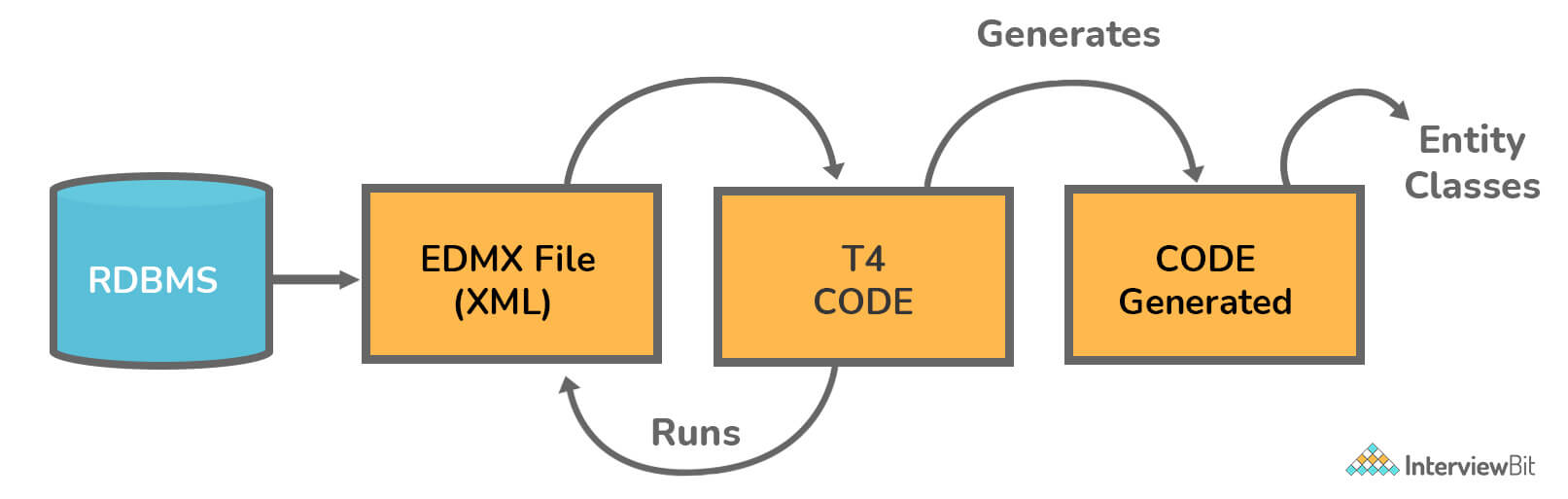
12. Explain CSDL, SSDL, and MSL sections in an Edmx file?
- CSDL: This stands for Conceptual Schema Definition Language. Basically, it's a conceptual abstraction that is exposed to the application. In this file, you will find a description of the model object.
- SSDL: This stands for Storage Schema Definition Language. In this section, we define the mapping to our RDBMS data structure.
- MSL: This stands for Mapping Schema Language. SSDL and CSDL are connected by it. It bridges the gap between the CSDL and SSDL or maps the model and the storage.
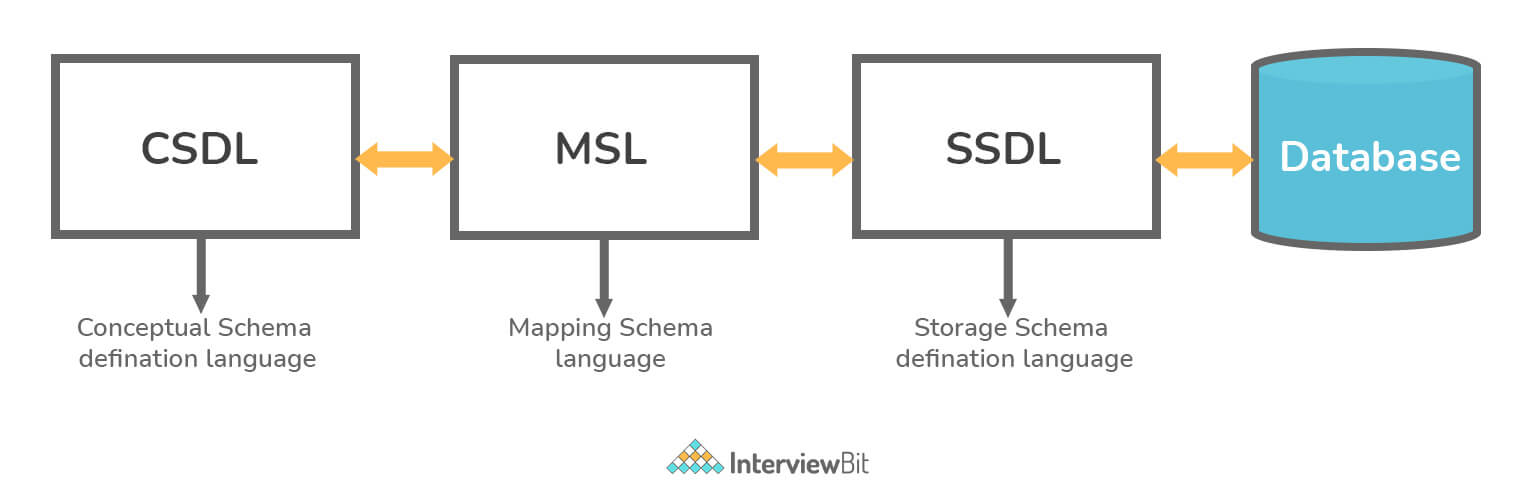
13. Explain the ways to increase the performance of EF.
Entity Framework's performance is enhanced by following these steps:
- Choose the right collection for data manipulation.
- Do not put all DB objects into one entity model.
- When the entity is no longer required, its tracking should be disabled and altered.
- Use pre-generating Views to reduce response time for the first request.
- Don't fetch all fields unless needed.
- Whenever possible, avoid using Views and Contains.
- Bind data to a grid or paging only by retrieving the number of records needed.
- Optimize and debug LINQ queries.
- Whenever possible, use compiled queries.
14. Difference Between
Pessimistic Approach and Optimistic Approach :
Pessimistic
Approach |
Optimistic
Approach |
It
locks records so that selected record for update will not be changed meantime
by another user |
It
doesn’t lock the records as it ensures record wasn’t changed in time between
SELECT & SUBMIT operations. |
The
conflicts between transactions are very large in this approach |
The
conflicts between transactions are less as compared to pessimistic approach. |
The
synchronization of transactions is conducted in start phase of life cycle of
execution of a transaction |
The
synchronization of transactions is conducted in later phase or gets delayed
in execution of a transaction |
It
is simple in designing and in programming. |
It
is more complex in designing and managing deadlocks’ risk |
It
has a higher storage cost |
It
has a relatively lower storage cost as compared to pessimistic approach |
It
has a lower degree of concurrency |
It
has a high degree of concurrency |
This
approach is found to use where there are more transaction conflicts |
This
approach is found to use where there are fewer transaction conflicts or very
rare |
The
flow of transaction phases: Validate
-> Read -> Compute -> Write |
The
flow of transaction phases: Read
-> Compute -> Validate -> Write |
It
helps in protecting the system from the concurrency conflict |
It
allows the conflict to happen |
It
is suitable for a small database or a table which has less records |
It
is suitable for a large database or has more records |
15. What do you mean by the migration history table in Entity Framework?
EF6's Migration's history table (__MigrationHistory) is basically a database table that is used to store data about migrations applied to a database by Code First Migrations. A table like this is created when the first migration is applied to the database. In Entity Framework 5 this table was a system table if the application used Microsoft Sql Server database. This has changed in Entity Framework 6 however and the migrations history table is no longer marked a system table.
16. Explain how EF supports transactions.
The SaveChanges() method in EF always wraps any operation involving inserting, updating, or deleting data into a transaction. Hence, you do not have to explicitly open the transaction scope.
Entity Framework Interview Questions for Experienced
17. Write difference between LINQ and Entity Framework.
LINQ | Entity Framework |
---|---|
In order to operate, LINQ relies only on SQL Server Databases. | In order to operate, the entity framework relies on several databases including SQL Server, Oracle, MYSQL, DB2, etc. |
It generates a .dbml to maintain the relationship. | In this case, an .edmx file is generated first, then an .edmx file is maintained using three separate files- .csdl, .msl, and .ssdl. |
DataContext enables you to query data. | ObjectContext, DbContext, and EntitySQL can all be used to query data. |
Complex types are not supported. | Complex types are supported. |
A database is not created from the model. | A database can be created from the model. |
Application is developed more quickly using SQL Server. | Applications are developed more quickly using SQL Server and other databases like MYSQL, Oracle, DB2, etc. |
It consists of a tightly coupled mechanism. | It consists of a loosely coupled mechanism. |
Only one-to-one mappings are allowed. | One-to-one, one-to-many & many-to-many mappings are allowed. |
It displays rapid development. | It takes longer to develop than LINQ, but it provides more capabilities. |
18. Explain the term dbcontext and dbset.
DbSet: An entity set is represented by a DbSet class that can be used for creating, reading, updating, and deleting operations on it. Those DbSet type properties, which map to database tables and views, must be included in the context class (derived from DbContext).
DbContext: It is considered an essential class in EF API that bridges the gap between an entity or domain class and the database. Communication with the database is its primary responsibility.
19. Difference between ADO.Net and Entity Framework.
Below are the differences between Aadonet and Entity Framework:
- A few data layer codes are created by Ado.Net that Entity Framework doesn't create.
- Entity Framework, unlike ADO.Net, generates code for intermediate layers, data access layers, and mappings automatically. This results in a reduction in development time.
- On a performance basis, ADO.Net is more efficient and faster than Entity Framework.
20. Explain the role of Pluralize and Singularize in the entity framework.
Objects in Entity Framework are primarily assigned names using Pluralize and Singularize. This feature is available when adding a .edmx file. Entity Framework automatically assigns the Singular or Plural coding conventions when using this feature. In convention names, an additional 's' is added if there is more than one record in the object.
21. What is the difference between Dapper and Entity Framework?
.NET developers are allowed to work with relational data using domain-specific objects by object-relational mappers such as Entity Framework (EF) and Dapper. Performance-wise, Dapper is the King of Micro ORMs.
- Dapper: A simple micro ORM, Dapper is considered a powerful system used for data access in the .NET world. As a means to address and open-source their issues, the Stack Overflow team created Dapper. Adding this NuGet library to your .NET project allows you to perform database operations. In terms of speed, it is the king of Micro ORMs and is almost as fast as using raw ADO.NET data readers.
- Entity Framework: It is a set of .NET APIs used in software development for performing data access. It is Microsoft's official tool for accessing data.
Comparison
- According to NuGet downloads and performance, Dapper is the world's most popular Micro ORM. In contrast, Entity Framework is significantly slower than Dapper.
- In comparison to other ORMs, such as the Entity Framework, Dapper does not generate as much SQL, but it does an excellent job mapping from database columns to CLR properties.
- Since Dapper uses RAW SQL, it can be difficult to code, especially when multiple relationships are involved, but when a lot of data is involved and performance matters, it is worth the effort.
- Since Dapper uses IDbConnection, developers can execute SQL queries to the database directly rather than put data in other objects as they do in Entity Framework.
22. Explain POCO Classes in EF.
POCO stands for 'Plain Old CLR Objects'. Yet, it does not mean these classes are plain or old. A POCO class is defined as a class that contains no reference to the EF Framework or the .NET Framework at all. In EF applications, Poco entities are known as available domain objects.
23. Optimistic vs. Pessimistic locking?
Optimistic Locking is a strategy where you read a record, take note of a version number (other methods to do this involve dates, timestamps or checksums/hashes) and check that the version hasn't changed before you write the record back. When you write the record back you filter the update on the version to make sure it's atomic. (i.e. hasn't been updated between when you check the version and write the record to the disk) and update the version in one hit.
If the record is dirty (i.e. different version to yours) you abort the transaction and the user can re-start it.
Pessimistic locking is an approach of concurrency control algorithms in which the transaction is delayed if there is a conflict with each other at some point of time in the future. It locks the database’s record for update access and other users can only access record as read-only or have to wait for a record to be ‘unlocked’. Programming an app with a pessimistic concurrency approach can be more complicated and complex in managing because of deadlocks’ risk.
24. Explain database concurrency and the way to handle it.
Database concurrency in EF means that multiple users can simultaneously modify the same data in one database. Concurrency controls help safeguard data consistency in situations like these.
Optimistic locking is usually used to handle database concurrency. We must first right-click on the EDMX designer and then change the concurrency mode to Fixed in order to implement locking. With this change, if there is a concurrency issue, we will receive a positive concurrency exception error.
26. What do you mean by lazy loading, eager loading and explicit loading?
Lazy Loading:
This process delays the loading of related objects until they are needed. During lazy loading, only the objects needed by the user are returned, whereas all other related objects are only returned when needed.
public partial class SchoolDBEntities : DbContext{public SchoolDBEntities(): base("name=SchoolDBEntities"){this.Configuration.LazyLoadingEnabled = true;}protected override void OnModelCreating(DbModelBuilder modelBuilder){}}
User usr = dbContext.Users.FirstOrDefault(a => a.UserId == userId);
UserDeatils ud = usr.UserDetails; // UserDetails are loaded here
- Use Lazy Loading when you are using one-to-many collections.
- Use Lazy Loading when you are sure that you are not using related entities instantly.
User usr = dbContext.Users.Include(a => a.UserDetails).FirstOrDefault(a => a.UserId == userId);
User usr = dbContext.Users.Include(a => a.UserDetails.Select(ud => ud.Address)).FirstOrDefault(a => a.UserId == userId);
- Use Eager Loading when the relations are not too much. Thus, Eager Loading is a good practice to reduce further queries on the Server.
- Use Eager Loading when you are sure that you will be using related entities with the main entity everywhere.
There are options to disable Lazy Loading in an Entity Framework. After turning Lazy Loading off, you can still load the entities by explicitly calling the Load method for the related entities. There are two ways to use Load method Reference (to load single navigation property) and Collection (to load collections), as shown below. When the Load method is used, explicit loading can be achieved in EF6.
using (var context = new SchoolContext()){ var student = context.Students .Where(s => s.FirstName == "Bill") .FirstOrDefault<Student>();
context.Entry(student).Reference(s => s.StudentAddress).Load(); // loads StudentAddress context.Entry(student).Collection(s => s.StudentCourses).Load(); // loads Courses collection }
- When you have turned off Lazy Loading, use Explicit loading when you are not sure whether or not you will be using an entity beforehand.
27. Explain Complex Type in Entity Framework.
28. What do you mean by Micro ORM?
A Micro ORM (Object-Relational Mapping) is a lightweight and simple ORM tool that provides a basic set of functionalities to map database objects to object-oriented programming languages. Micro ORM focuses on simplicity, performance, and flexibility.
Unlike full-fledged ORM frameworks, Micro ORM tools offer a minimalistic set of features that are easy to learn and use. They often provide basic CRUD (Create, Read, Update, Delete) operations and rely on convention-over-configuration to reduce boilerplate code. Micro ORM tools may not provide all the features and optimizations that full-fledged ORM frameworks offer.
Examples of Micro ORM tools include Dapper for .NET, SQLObject for Python, and MyBatis for Java.
29. Explain EF Data access Architecture.
There are two types of Data Access Architecture supported by the ADO.NET Framework:
- Disconnected data access: Disconnected data access is possible with the Data Adapter object. Datasets work independently of databases, and the data can be edited.
- Connected data access: A Data Reader object of a Data Provider allows you to access linked data. Data can be accessed quickly, but editing is not permitted.
30. What do you mean by SQL injection attack?
SQL injection is a method that hackers use to access sensitive information from an organization's database. This application-layer attack is the result of inappropriate coding in our applications, allowing hackers to inject SQL statements into your SQL code.
The most common cause of SQL Injection is that user input fields allow SQL statements to pass through and directly query the database. ADO.NET Data Services queries are commonly affected by SQL Injection issues.
31. What is the best way to handle SQL injection attacks in Entity Framework?
The injection-proof nature of Entity Framework lies in the fact that it generates parameterized SQL commands that help prevent our database from SQL injections.
By inserting some malicious inputs into queries and parameter names, one can generate a SQL injection attack in Entity SQL syntax. It is best to never combine user inputs with Entity SQL commands text to prevent or avoid this problem.
32. Write the namespace that is used to include .NET Data provider for SQL server in .NET code.
NET Data Provider for SQL Server is included in .NET code by using the namespace System.Data.SqlClient.
33. What do you mean by DbEntityEntry Class in EF?
An important class, DbEntityEntry helps you retrieve a variety of information about an entity. DbContext offers the Entry method for retrieving an instance of DBEntityEntry of a specific entity.
Example:
DbEntityEntry studentEntry = dbcontext.Entry(entity);
You can access the entity state, as well as the current and original values of all properties of an entity using the DbEntityEntry. EntityState can be set using the DbEntityEntry, as shown below.
context.Entry(student).State = System.Data.Entity.EntityState.Modified;
34) What can we do to improve the performance of the Entity Framework?
We can use the following ways to improve the performance of the Entity Framework:
- We can use compiled queries whenever required.
- We must avoid the use of Views and Contains.
- We can disable and alter tracking for the entity when it is not needed.
- We can Debug and Optimize the LINQ query.
- If not obligatory, we must try to evade fetching all the fields.
- We should retrieve only the desired number of records when binding data to the grid.
35) Tracking vs. No-Tracking?
Tracking behavior controls if Entity Framework Core will keep information about an entity instance in its change tracker. If an entity is tracked, any changes detected in the entity will be persisted to the database during SaveChanges();
No tracking queries are useful when the results are used in a read-only scenario. They're quicker to execute because there's no need to set up the change tracking information. If you don't need to update the entities retrieved from the database, then a no-tracking query should be used.
var blogs = context.Blogs.AsNoTracking().ToList();
36) SQL Queries Entity Framework?
Entity Framework Core allows you to drop down to SQL queries when working with a relational database. SQL queries are useful if the query you want can't be expressed using LINQ, or if a LINQ query causes EF to generate inefficient SQL.
FromSql:
FromSql is useful for querying entities defined in your model. FromSqlRaw, which allows interpolating variable data directly into the SQL string and dynamically construct your SQL
var blogs = context.Blogs.FromSql($"SELECT * FROM dbo.Blogs").ToList().
var columnName = "Url"; var columnValue = new SqlParameter("columnValue", "http://SomeURL");
var blogs = context.Blogs.FromSqlRaw($"SELECT * FROM [Blogs] WHERE {columnName} = @columnValue", columnValue) .ToList();
SqlQuery
Allows you to easily query for scalar, non-entity types via SQL
var ids = context.Database.SqlQuery<int>($"SELECT [BlogId] FROM [Blogs]").ToList();
typically for modifying data in the database or calling a stored procedure which doesn't
ExecuteSqlRaw allows for dynamic construction of SQL queries
using (var context = new BloggingContext())
{
var rowsModified = context.Database.ExecuteSql($"UPDATE [Blogs] SET [Url] = NULL");
}
37) User-defined function mapping in Entity Framework?
EF Core allows for using user-defined SQL functions in queries. To do that, the functions need to be mapped to a CLR method during model configuration.
CREATE FUNCTION dbo.CommentedPostCountForBlog(@id int)
RETURNS int
AS
BEGIN
RETURN (SELECT COUNT(*) FROM [Posts] AS [p]
WHERE ([p].[BlogId] = @id) AND ((
SELECT COUNT(*) FROM [Comments] AS [c]
WHERE [p].[PostId] = [c].[PostId]) > 0));
END
This function definition can now be associated with user-defined function in the model configuration:
modelBuilder.HasDbFunction(typeof(BloggingContext).GetMethod(nameof(ActivePostCountForBlog), new[] { typeof(int) })).HasName("CommentedPostCountForBlog");
38) Code First Model class configuration??
Code First gives you two ways to add these configurations to your classes. One is using simple attributes called DataAnnotations, and the second is using Code First’s Fluent API, which provides you with a way to describe configurations imperatively, in code.
https://www.learnentityframeworkcore.com/configuration/fluent-api
39) Working with Stored Procedure in Entity Framework Core?
40) EF Object context vs DB context?
In Entity Framework, both ObjectContext and DbContext are classes that provide a way to interact with a database. However, DbContext is a newer and simpler API that was introduced in Entity Framework 4.1 as a replacement for ObjectContext.
The main differences between ObjectContext and DbContext are as follows:
- ObjectContext is the older API that is still available in Entity Framework, while DbContext is the newer, preferred API that provides a simpler and more intuitive interface.
- DbContext is a lightweight wrapper around ObjectContext, providing a simplified way of working with the Entity Framework.
- DbContext provides a simpler and more intuitive API for working with entities, while ObjectContext requires more verbose and complicated code.
- DbContext also provides built-in support for Code First, which is a way of creating a database from code, while ObjectContext requires more manual configuration.
- DbContext supports dependency injection, making it easier to use in a variety of application architectures, while ObjectContext does not.
- Finally, DbContext is faster and more efficient than ObjectContext, as it is optimized for performance and resource usage.
Overall, while ObjectContext is still available and supported in Entity Framework, DbContext is generally considered to be the preferred way of working with the Entity Framework, especially in newer applications. It provides a simpler, more intuitive API, supports Code First, and is optimized for performance and resource usage.
No comments:
Post a Comment